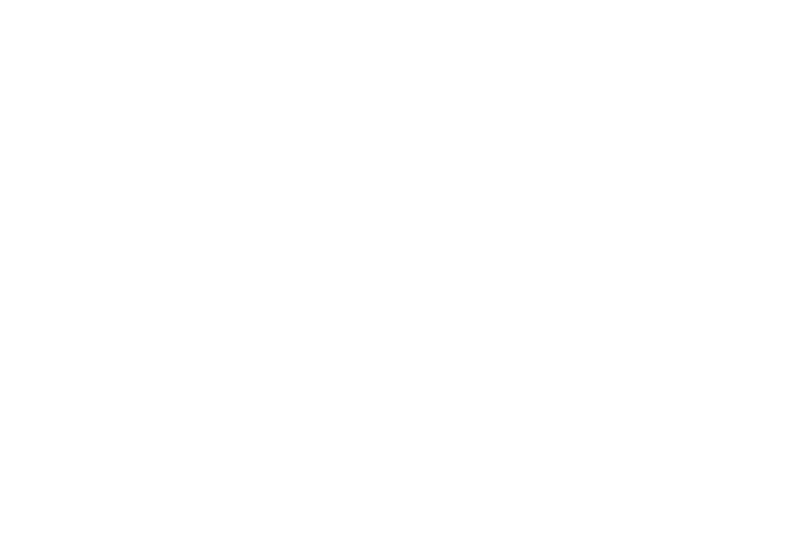
How to send a concatenated/long SMS
When sending a SMS you have to consider the maximum length of the SMS. The maximum length depends on the character set you use. For 7-bit GSM characters the maximum length is 160 characters and for Unicode the maximum length is 70 characters. Sometimes you want to send longer SMS to your customers. In this tutorial we will explain how you can do that.
7-bit GSM characters vs. Unicode
The 7-bit GSM character set is a limited set of characters you can send. The following characters are available in this character set:
@£$¥èéùìòÇØøÅåΔ_ΦΓΛΩΠΨΣΘΞ^{}[~]|€ÆæßÉ!"#¤%&'()*+,-./0123456789:;<=>?¡
ABCDEFGHIJKLMNOPQRSTUVWXYZÄÖÑܧ¿abcdefghijklmnopqrstuvwxyzäöñüà
When you want to send other characters, e.g. Chinese, you have to use Unicode. Our API will simply detect if the body is 7-bit GSM or not, so you don’t have to do anything for that.
Concatenated/long SMS
The maximum length for normal SMS messages is 160 characters and for Unicode 70 characters. If you want to send longer messages, you will need to split up the body of the message by sending each body part of the message separately and adding a User Data Header (UDH). See also GSM specification for more information. The maximum length for normal messages will then be 153 characters per body part and for Unicode 67 characters per body part.
With the Silverstreet API there are 2 ways to send such long SMS messages.
- Using the simple SMS API function
- Using the advanced SMS API function
Simple SMS API function
When you use our Simple SMS API function, the API will split up the message for you and generates the UDH. It will automatically detect if the message is Unicode and split up into maximum 9 concatenated parts.
Example of a long message using our Simple SMS API:
<?php
$body = 'This is a long message, This is a long message, This is a long message, This is a long message, This is a long message, This is a long message, This is a long message.';
$recipient = '316987654321';
$sender = '316123456789';
$sms = $client->createSms($body, $recipient, $sender);
$sms->sendSimple();
?>
Advanced SMS API function
With the advanced SMS API function you will have to create the UDH yourself. The UDH is a set of bytes, for concatenated SMS you will have to setup the UDH as follows:
1st byte | This byte specifies the length of the UDH. |
2nd byte | This byte is the Identity Element Identifier (IEI). For concatenated SMS we can set this to `00` or `08`. The value `08` is mainly interesting when you send many (> 256) concatenated SMS to the same number. |
3rd byte | This byte specifies the length of the IEI. When you have set `00` for the IEI, you will have to set this length to ’03`, when you have set `08` for the IEI you will have to set this length to `04`. |
4th byte | This is the reference number for this concatenated SMS. All parts of this concatenated SMS must have the same reference so the phone knows which parts belong to each other. When you have set for the IEI the value `00`, the reference can have a value between `00` and `FF`. When you have set for the IEI the value `08`, the reference can have a value between `0000` and `FFFF`. |
5th byte | This byte specifies how many message parts there are. Most phones support up to 9 message parts. When you message is split into 7 parts, you will have to set the value `07`. |
6th byte | This byte specifies the part number. When it is for example the first part, you will have to set the value `01`. |
So an example UDH for a concatenated SMS with 7 parts and this is part number 5 is (for readability we added white spaces in the example but you have to remove them when sending to the API):
05 00 03 A0 07 05
Example for sending one message part:
<?php
$body = 'This is a long message, This is a long message, This is a long message, This is a long message, This is a long message, This is a long message, This is a';
$recipient = '316987654321';
$sender = '316123456789';
$udh = '050003A00201';
$sms = $client->createSms($body, $recipient, $sender);
$sms->setUdh($udh);
$sms->send();
?>